components
Tailwind CSS Carousel
Utilize the carousel component to navigate through various elements and images with customized controls, indicators, intervals, and settings.
Class Name | Type | Description |
---|---|---|
carousel | Component | A wrapper for a collection of slides. It must contain the overflow-hidden class, it is needed for the slider to work correctly. |
carousel-body | Component | Slides container. |
carousel-slide | Component | Single slide. |
carousel-prev | Component | Previous slide button. |
carousel-next | Component | Next slide button. |
carousel-pagination | Component | Base class for pagination container. |
carousel-dot | Component | Base class for pagination indicators. |
active | Modifier | When it is applied, the slide becomes visible. |
carousel-active:{tw-utility-class} | Modifier | It sets Tailwind classes when the active slide is shown. |
Below example shows the default carousel with three slides.
<div id="carousel-1" data-carousel='{ "loadingClasses": "opacity-0" }' class="relative w-full">
<div class="carousel">
<div class="carousel-body opacity-0">
<!-- Slide 1 -->
<div class="carousel-slide">
<div class="bg-base-300/60 flex h-full justify-center p-6">
<span class="self-center text-2xl sm:text-4xl">First slide</span>
</div>
</div>
<!-- Slide 2 -->
<div class="carousel-slide">
<div class="bg-base-300/80 flex h-full justify-center p-6">
<span class="self-center text-2xl sm:text-4xl">Second slide</span>
</div>
</div>
<!-- Slide 3 -->
<div class="carousel-slide">
<div class="bg-base-300 flex h-full justify-center p-6">
<span class="self-center text-2xl sm:text-4xl">Third slide</span>
</div>
</div>
</div>
</div>
<!-- Previous Slide -->
<button type="button" class="carousel-prev">
<span class="size-9.5 bg-base-100 flex items-center justify-center rounded-full shadow">
<span class="icon-[tabler--chevron-left] size-5 cursor-pointer rtl:rotate-180"></span>
</span>
<span class="sr-only">Previous</span>
</button>
<!-- Next Slide -->
<button type="button" class="carousel-next">
<span class="sr-only">Next</span>
<span class="size-9.5 bg-base-100 flex items-center justify-center rounded-full shadow">
<span class="icon-[tabler--chevron-right] size-5 cursor-pointer rtl:rotate-180"></span>
</span>
</button>
</div>
Below example shows the carousel with indicators.
<div id="carousel-2" data-carousel='{ "loadingClasses": "opacity-0" }' class="relative w-full" >
<div class="carousel">
<div class="carousel-body opacity-0">
<!-- Slide 1 -->
<div class="carousel-slide">
<div class="bg-base-300/60 flex h-full justify-center p-6">
<span class="self-center text-2xl sm:text-4xl">First slide</span>
</div>
</div>
<!-- Slide 2 -->
<div class="carousel-slide">
<div class="bg-base-300/80 flex h-full justify-center p-6">
<span class="self-center text-2xl sm:text-4xl">Second slide</span>
</div>
</div>
<!-- Slide 3 -->
<div class="carousel-slide">
<div class="bg-base-300 flex h-full justify-center p-6">
<span class="self-center text-2xl sm:text-4xl">Third slide</span>
</div>
</div>
</div>
<div class="carousel-pagination">
<span class="carousel-dot carousel-active:bg-primary carousel-active:border-primary"></span>
<span class="carousel-dot carousel-active:bg-primary carousel-active:border-primary"></span>
<span class="carousel-dot carousel-active:bg-primary carousel-active:border-primary"></span>
</div>
</div>
<!-- Previous Slide -->
<button type="button" class="carousel-prev">
<span class="size-9.5 bg-base-100 flex items-center justify-center rounded-full shadow">
<span class="icon-[tabler--chevron-left] size-5 cursor-pointer rtl:rotate-180"></span>
</span>
<span class="sr-only">Previous</span>
</button>
<!-- Next Slide -->
<button type="button" class="carousel-next">
<span class="sr-only">Next</span>
<span class="size-9.5 bg-base-100 flex items-center justify-center rounded-full shadow">
<span class="icon-[tabler--chevron-right] size-5 cursor-pointer rtl:rotate-180"></span>
</span>
</button>
</div>
Below example shows carousel with progress.
<div id="carousel-3" data-carousel='{ "loadingClasses": "opacity-0" }' class="relative w-full" id="carousel-progress" >
<div class="carousel rounded-none">
<div class="carousel-body opacity-0">
<!-- Slide 1 -->
<div class="carousel-slide">
<div class="bg-base-200/40 flex h-full justify-center p-6">
<span class="self-center text-2xl sm:text-4xl">First slide</span>
</div>
</div>
<!-- Slide 2 -->
<div class="carousel-slide">
<div class="bg-base-200/60 flex h-full justify-center p-6">
<span class="self-center text-2xl sm:text-4xl">Second slide</span>
</div>
</div>
<!-- Slide 3 -->
<div class="carousel-slide">
<div class="bg-base-300/60 flex h-full justify-center p-6">
<span class="self-center text-2xl sm:text-4xl">Third slide</span>
</div>
</div>
<!-- Slide 4 -->
<div class="carousel-slide">
<div class="bg-base-300/80 flex h-full justify-center p-6">
<span class="self-center text-2xl sm:text-4xl">Fourth slide</span>
</div>
</div>
<!-- Slide 5 -->
<div class="carousel-slide">
<div class="bg-base-300 flex h-full justify-center p-6">
<span class="self-center text-2xl sm:text-4xl">Fifth slide</span>
</div>
</div>
</div>
<div class="carousel-pagination space-x-0 justify-start top-0">
<span class="w-1/5 h-1 hidden carousel-active:block carousel-active:bg-primary"></span>
<span class="w-2/5 h-1 hidden carousel-active:block carousel-active:bg-primary"></span>
<span class="w-3/5 h-1 hidden carousel-active:block carousel-active:bg-primary"></span>
<span class="w-4/5 h-1 hidden carousel-active:block carousel-active:bg-primary"></span>
<span class="w-full h-1 hidden carousel-active:block carousel-active:bg-primary"></span>
</div>
</div>
<!-- Previous Slide -->
<button type="button" class="carousel-prev">
<span class="size-9.5 bg-base-100 flex items-center justify-center rounded-full shadow">
<span class="icon-[tabler--chevron-left] size-5 cursor-pointer rtl:rotate-180"></span>
</span>
<span class="sr-only">Previous</span>
</button>
<!-- Next Slide -->
<button type="button" class="carousel-next">
<span class="sr-only">Next</span>
<span class="size-9.5 bg-base-100 flex items-center justify-center rounded-full shadow">
<span class="icon-[tabler--chevron-right] size-5 cursor-pointer rtl:rotate-180"></span>
</span>
</button>
</div>
Below example shows the carousel with images.
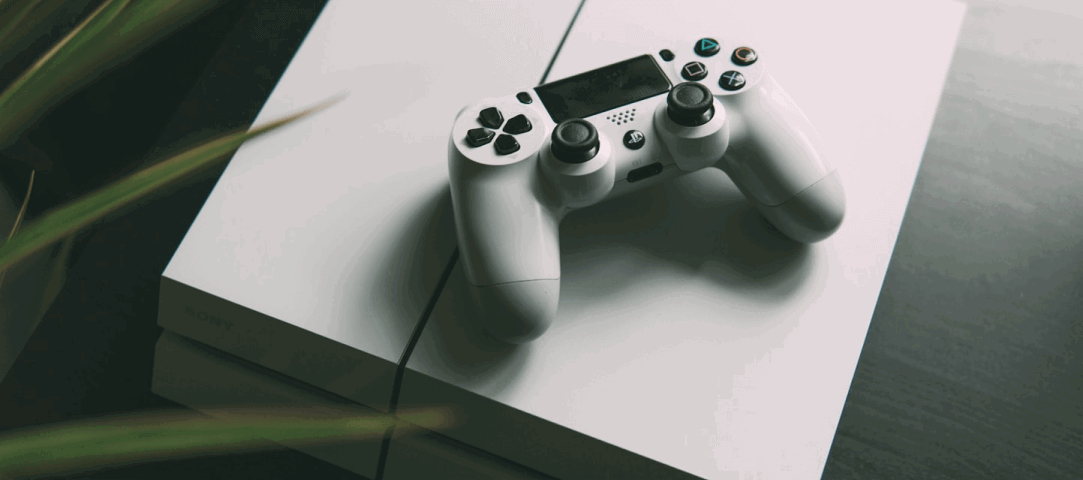
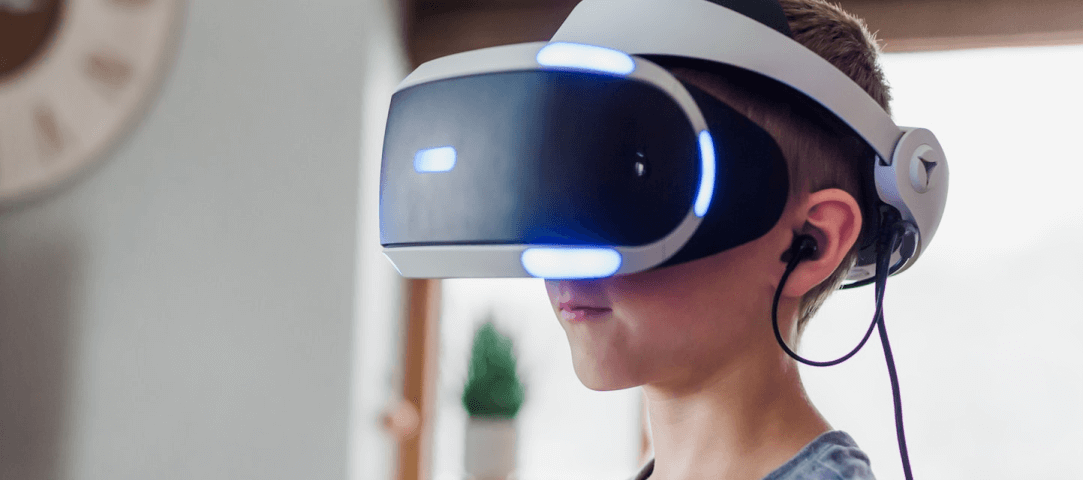
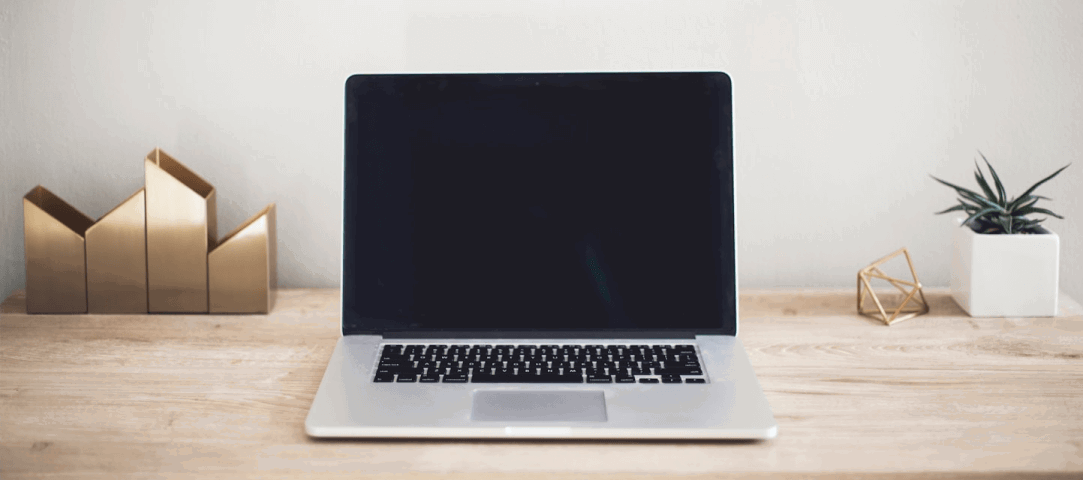
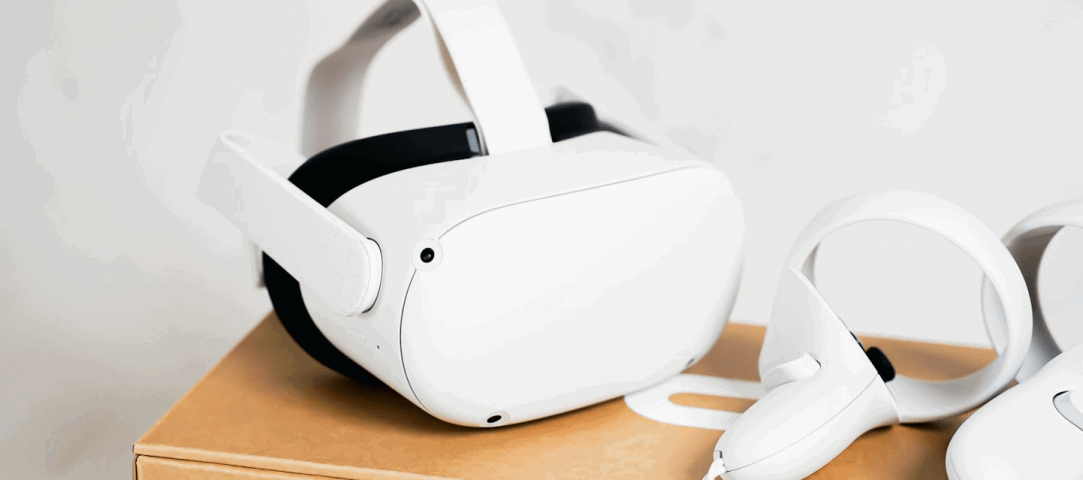
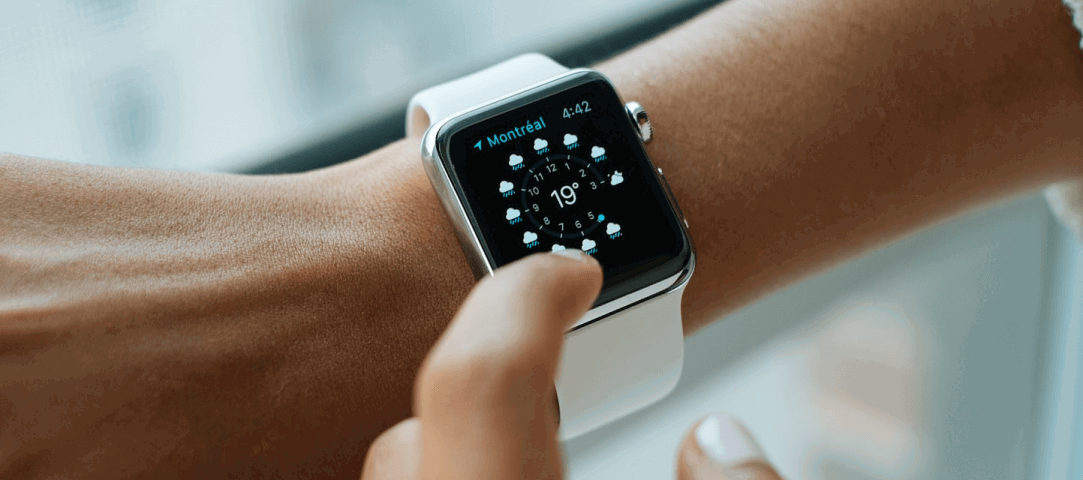
<div id="carousel-4" data-carousel='{ "loadingClasses": "opacity-0" }' class="relative w-full">
<div class="carousel">
<div class="carousel-body opacity-0">
<!-- Slide 1 -->
<div class="carousel-slide">
<div class="flex h-full justify-center">
<img src="https://cdn.flyonui.com/fy-assets/components/carousel/image-22.png" class="size-full object-cover" alt="game" />
</div>
</div>
<!-- Slide 2 -->
<div class="carousel-slide">
<div class="flex h-full justify-center">
<img src="https://cdn.flyonui.com/fy-assets/components/carousel/image-15.png" class="size-full object-cover" alt="vrbox" />
</div>
</div>
<!-- Slide 3 -->
<div class="carousel-slide">
<div class="flex h-full justify-center">
<img src="https://cdn.flyonui.com/fy-assets/components/carousel/image-16.png" class="size-full object-cover" alt="laptop" />
</div>
</div>
<!-- Slide 4 -->
<div class="carousel-slide">
<div class="flex h-full justify-center">
<img src="https://cdn.flyonui.com/fy-assets/components/carousel/image-8.png" class="size-full object-cover" alt="VRBox" />
</div>
</div>
<!-- Slide 5 -->
<div class="carousel-slide">
<div class="flex h-full justify-center">
<img src="https://cdn.flyonui.com/fy-assets/components/carousel/image-23.png" class="size-full object-cover" alt="iwatch" />
</div>
</div>
</div>
</div>
<!-- Previous Slide -->
<button type="button" class="carousel-prev">
<span class="size-9.5 bg-base-100 flex items-center justify-center rounded-full shadow">
<span class="icon-[tabler--chevron-left] size-5 cursor-pointer rtl:rotate-180"></span>
</span>
<span class="sr-only">Previous</span>
</button>
<!-- Next Slide -->
<button type="button" class="carousel-next">
<span class="sr-only">Next</span>
<span class="size-9.5 bg-base-100 flex items-center justify-center rounded-full shadow">
<span class="icon-[tabler--chevron-right] size-5 cursor-pointer rtl:rotate-180"></span>
</span>
</button>
</div>
Below example shows the carousel with thumbnails.
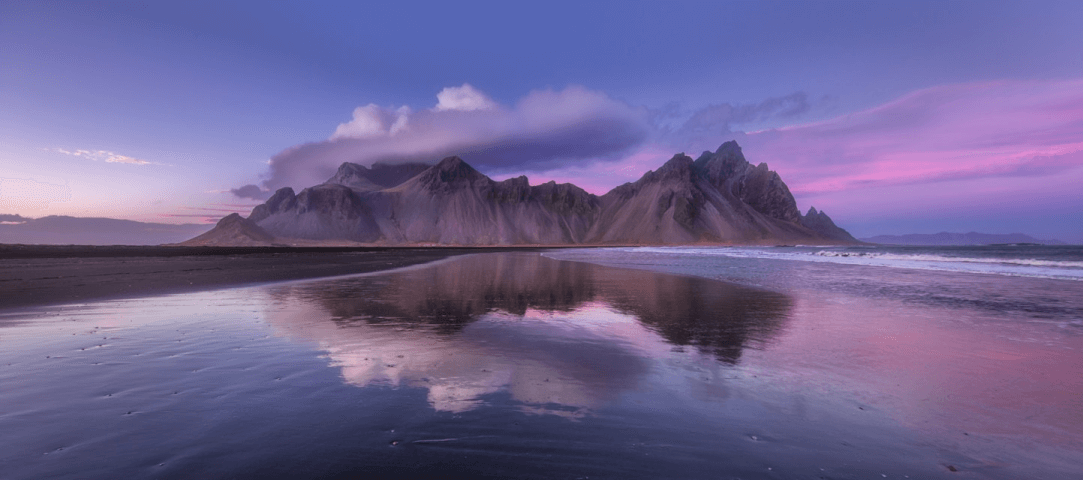
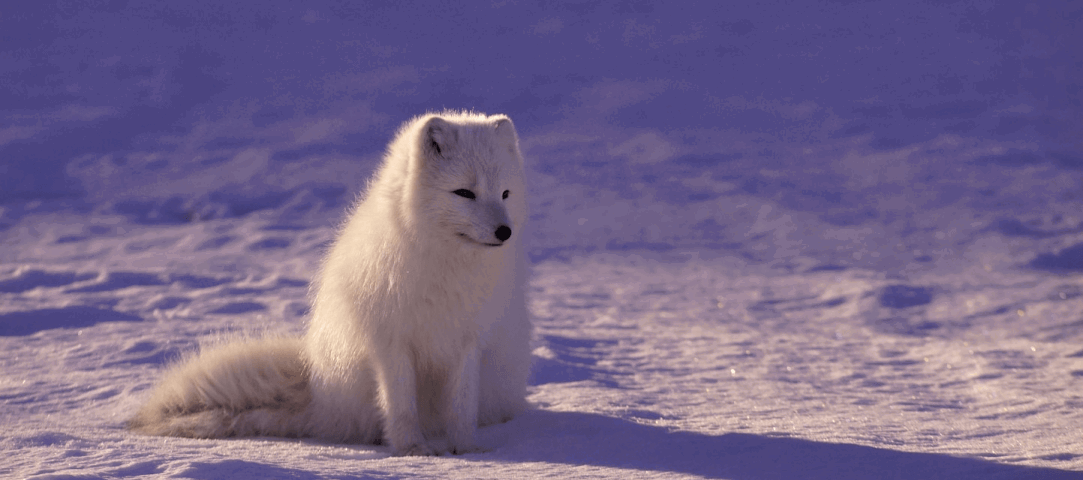
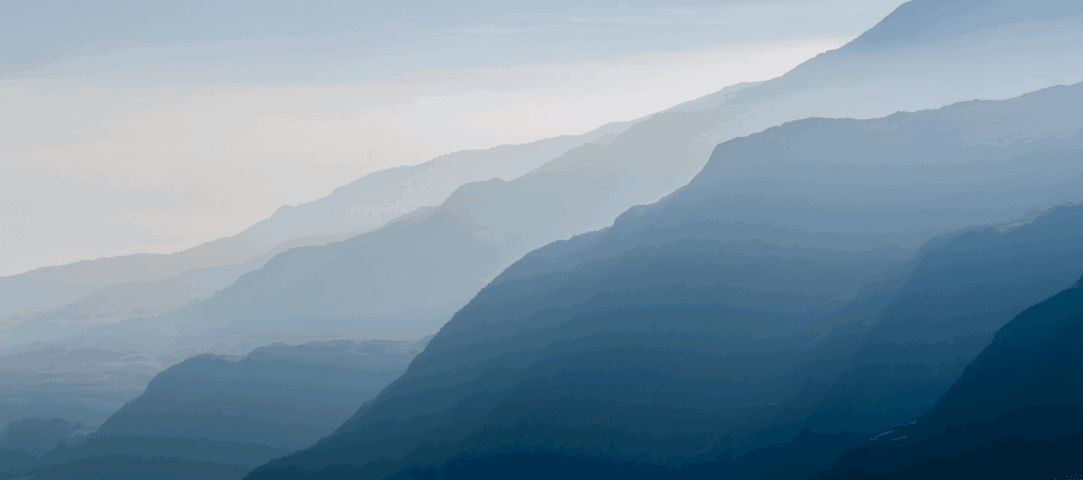
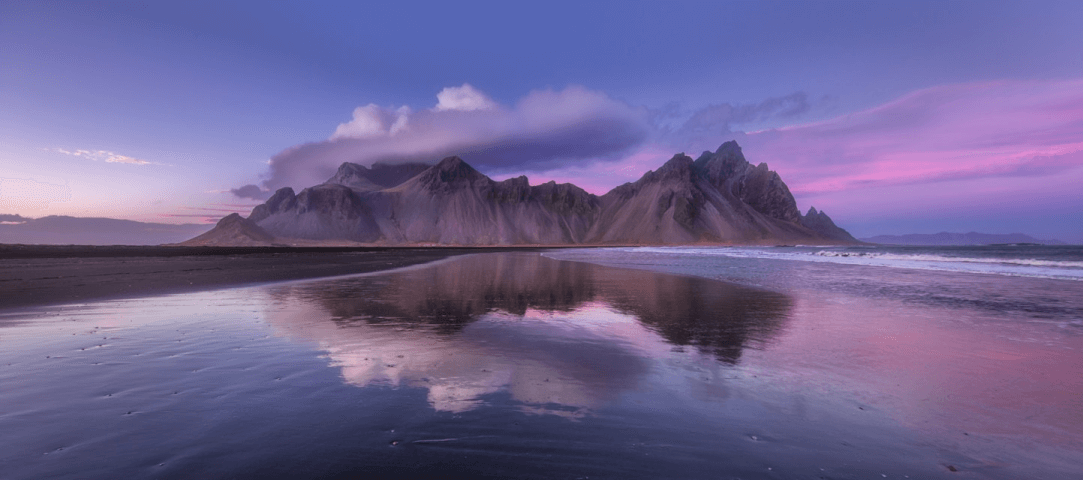
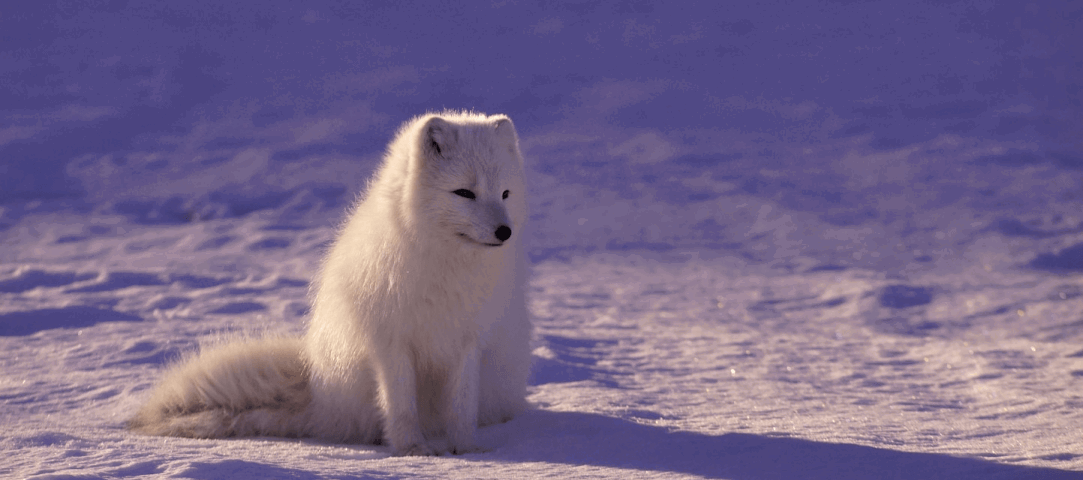
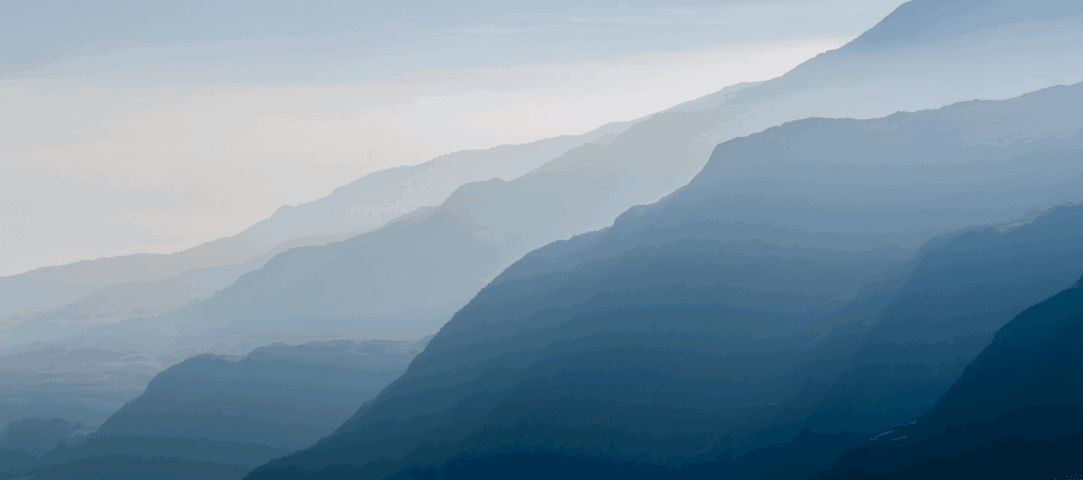
<div id="carousel-5" data-carousel='{ "loadingClasses": "opacity-0" }' class="relative w-full">
<div class="carousel h-96">
<div class="carousel-body h-3/4 opacity-0">
<!-- Slide 1 -->
<div class="carousel-slide">
<div class="flex size-full justify-center">
<img src="https://cdn.flyonui.com/fy-assets/components/carousel/image-21.png" class="size-full object-cover" alt="mountain" />
</div>
</div>
<!-- Slide 2 -->
<div class="carousel-slide">
<div class="flex size-full justify-center">
<img src="https://cdn.flyonui.com/fy-assets/components/carousel/image-14.png" class="size-full object-cover" alt="sand" />
</div>
</div>
<!-- Slide 3 -->
<div class="carousel-slide">
<div class="flex size-full justify-center">
<img src="https://cdn.flyonui.com/fy-assets/components/carousel/image-7.png" class="size-full object-cover" alt="cloud" />
</div>
</div>
</div>
<div class="carousel-pagination bg-base-100 bottom-0 z-[1] h-1/4 justify-between gap-2 space-x-0 pt-2 overflow-y-auto">
<img
src="https://cdn.flyonui.com/fy-assets/components/carousel/image-21.png"
class="carousel-active:opacity-100 grow object-cover opacity-30"
alt="mountain"
/>
<img
src="https://cdn.flyonui.com/fy-assets/components/carousel/image-14.png"
class="carousel-active:opacity-100 grow object-cover opacity-30"
alt="sand"
/>
<img
src="https://cdn.flyonui.com/fy-assets/components/carousel/image-7.png"
class="carousel-active:opacity-100 grow object-cover opacity-30"
alt="cloud"
/>
</div>
</div>
<!-- Previous Slide -->
<button type="button" class="carousel-prev">
<span class="mb-15" aria-hidden="true">
<span class="size-9.5 bg-base-100 flex items-center justify-center rounded-full shadow">
<span class="icon-[tabler--chevron-left] size-5 cursor-pointer rtl:rotate-180"></span>
</span>
</span>
<span class="sr-only">Previous</span>
</button>
<!-- Next Slide -->
<button type="button" class="carousel-next">
<span class="sr-only">Next</span>
<span class="mb-15" aria-hidden="true">
<span class="size-9.5 bg-base-100 flex items-center justify-center rounded-full shadow">
<span class="icon-[tabler--chevron-right] size-5 cursor-pointer rtl:rotate-180"></span>
</span>
</span>
</button>
</div>
Assign the value of the data-carousel
attribute as an object. Within this object, set the currentIndex
option to index-number
for default active slide based on its index. By default, its value is 0
.
<div id="carousel-6" data-carousel='{ "loadingClasses": "opacity-0", "currentIndex": 1 }' class="relative w-full">
<div class="carousel">
<div class="carousel-body opacity-0">
<!-- Slide 1 -->
<div class="carousel-slide">
<div class="bg-base-300/60 flex h-full justify-center p-6">
<span class="self-center text-2xl sm:text-4xl">First slide</span>
</div>
</div>
<!-- Slide 2 -->
<div class="carousel-slide">
<div class="bg-base-300/80 flex h-full justify-center p-6">
<span class="self-center text-2xl sm:text-4xl">Second slide</span>
</div>
</div>
<!-- Slide 3 -->
<div class="carousel-slide">
<div class="bg-base-300 flex h-full justify-center p-6">
<span class="self-center text-2xl sm:text-4xl">Third slide</span>
</div>
</div>
</div>
</div>
<!-- Previous Slide -->
<button type="button" class="carousel-prev">
<span class="size-9.5 bg-base-100 flex items-center justify-center rounded-full shadow">
<span class="icon-[tabler--chevron-left] size-5 cursor-pointer rtl:rotate-180"></span>
</span>
<span class="sr-only">Previous</span>
</button>
<!-- Next Slide -->
<button type="button" class="carousel-next">
<span class="sr-only">Next</span>
<span class="size-9.5 bg-base-100 flex items-center justify-center rounded-full shadow">
<span class="icon-[tabler--chevron-right] size-5 cursor-pointer rtl:rotate-180"></span>
</span>
</button>
</div>
Set the data-carousel
attribute’s value as an object. Inside this object, configure the isAutoPlay
option to true
to enable auto-play in the carousel. By default, its value is false
.
In addition to isAutoPlay
, set the speed
option to number
within the configuration to determine the auto-play speed. The default value for this option is 4000
.
<div id="carousel-7" data-carousel='{ "loadingClasses": "opacity-0", "isAutoPlay": true, "speed": 1000 }' class="relative w-full" >
<div class="carousel">
<div class="carousel-body opacity-0">
<!-- Slide 1 -->
<div class="carousel-slide">
<div class="bg-base-300/60 flex h-full justify-center p-6">
<span class="self-center text-2xl sm:text-4xl">First slide</span>
</div>
</div>
<!-- Slide 2 -->
<div class="carousel-slide">
<div class="bg-base-300/80 flex h-full justify-center p-6">
<span class="self-center text-2xl sm:text-4xl">Second slide</span>
</div>
</div>
<!-- Slide 3 -->
<div class="carousel-slide">
<div class="bg-base-300 flex h-full justify-center p-6">
<span class="self-center text-2xl sm:text-4xl">Third slide</span>
</div>
</div>
</div>
</div>
<!-- Previous Slide -->
<button type="button" class="carousel-prev">
<span class="size-9.5 bg-base-100 flex items-center justify-center rounded-full shadow">
<span class="icon-[tabler--chevron-left] size-5 cursor-pointer rtl:rotate-180"></span>
</span>
<span class="sr-only">Previous</span>
</button>
<!-- Next Slide -->
<button type="button" class="carousel-next">
<span class="sr-only">Next</span>
<span class="size-9.5 bg-base-100 flex items-center justify-center rounded-full shadow">
<span class="icon-[tabler--chevron-right] size-5 cursor-pointer rtl:rotate-180"></span>
</span>
</button>
</div>
Within the data-carousel
attribute’s value, create an object. Inside this object, set the isInfiniteLoop
option to false
to deactivate the infinite looping of slides in the carousel. The default value for this option is true
.
<div id="carousel-8" data-carousel='{ "loadingClasses": "opacity-0", "isInfiniteLoop": false }' class="relative w-full">
<div class="carousel">
<div class="carousel-body opacity-0">
<!-- Slide 1 -->
<div class="carousel-slide">
<div class="bg-base-300/60 flex h-full justify-center p-6">
<span class="self-center text-2xl sm:text-4xl">First slide</span>
</div>
</div>
<!-- Slide 2 -->
<div class="carousel-slide">
<div class="bg-base-300/80 flex h-full justify-center p-6">
<span class="self-center text-2xl sm:text-4xl">Second slide</span>
</div>
</div>
<!-- Slide 3 -->
<div class="carousel-slide">
<div class="bg-base-300 flex h-full justify-center p-6">
<span class="self-center text-2xl sm:text-4xl">Third slide</span>
</div>
</div>
</div>
</div>
<!-- Previous Slide -->
<button type="button" class="carousel-prev">
<span class="size-9.5 bg-base-100 flex items-center justify-center rounded-full shadow">
<span class="icon-[tabler--chevron-left] size-5 cursor-pointer rtl:rotate-180"></span>
</span>
<span class="sr-only">Previous</span>
</button>
<!-- Next Slide -->
<button type="button" class="carousel-next">
<span class="sr-only">Next</span>
<span class="size-9.5 bg-base-100 flex items-center justify-center rounded-full shadow">
<span class="icon-[tabler--chevron-right] size-5 cursor-pointer rtl:rotate-180"></span>
</span>
</button>
</div>
PARAMETERS | DESCRIPTION | OPTIONS | DEFAULT VALUE |
---|---|---|---|
data-carousel | Activates a carousel by specifying on an element. | — | — |
:currentIndex | Specifies the index of the current slide initially (from 0 to slides quantity). | number | 0 |
:loadingClasses | Specifies which classes should be removed after the carousel is loaded. CSS classes should be separated with space. | string | opacity-0 |
:isAutoPlay | Enables autoplay. | boolean | false |
:speed | Autoplay animation speed. Available if isAutoplay: true . | number | 4000 |
:isInfiniteLoop | Enables infinite loop. | boolean | true |
The HSCarousel
object is contained within the global window
object.
METHOD | DESCRIPTION |
---|---|
PUBLIC METHODS | |
goToPrev() | Go to the previous slide. |
goToNext() | Go to the next slide. |
goTo(index) | Go to the slide by index. Starts from 0. |
recalculateWidth() | Recalculate the width of the carousel. |
STATIC METHODS | |
HSCarousel.getInstance(target) | Returns the element associated to the target .
|
Below, we demonstrate how to use the methods. Apply the ID to the carousel
container. To test the other method, copy the provided code into your console and click the button.
<div data-carousel='{ "loadingClasses": "opacity-0" }' class="relative w-full" id="carouselTarget">
<div class="carousel">
<div class="carousel-body opacity-0">
<!-- Slide 1 -->
<div class="carousel-slide">
<div class="bg-base-300/60 flex h-full justify-center p-6">
<span class="self-center text-2xl sm:text-4xl">First slide</span>
</div>
</div>
<!-- Slide 2 -->
<div class="carousel-slide">
<div class="bg-base-300/80 flex h-full justify-center p-6">
<span class="self-center text-2xl sm:text-4xl">Second slide</span>
</div>
</div>
<!-- Slide 3 -->
<div class="carousel-slide">
<div class="bg-base-300 flex h-full justify-center p-6">
<span class="self-center text-2xl sm:text-4xl">Third slide</span>
</div>
</div>
</div>
</div>
<!-- Previous Slide -->
<button type="button" class="carousel-prev">
<span class="size-9.5 bg-base-100 flex items-center justify-center rounded-full shadow">
<span class="icon-[tabler--chevron-left] size-5 cursor-pointer rtl:rotate-180"></span>
</span>
<span class="sr-only">Previous</span>
</button>
<!-- Next Slide -->
<button type="button" class="carousel-next">
<span class="sr-only">Next</span>
<span class="size-9.5 bg-base-100 flex items-center justify-center rounded-full shadow">
<span class="icon-[tabler--chevron-right] size-5 cursor-pointer rtl:rotate-180"></span>
</span>
</button>
</div>
<div><button id="go-to-2-slide" class="btn btn-primary">Go to slide 2</button></div>
<script>
window.addEventListener('load', function () {
const goTo2Btn = document.querySelector('#go-to-2-slide')
goTo2Btn.addEventListener('click', () => {
const carousel = new HSCarousel(document.querySelector('#carouselTarget'))
carousel.goTo(1)
})
})
</script>
Go to certain slide by button click example (public method).
// This method is used in above example.
const goTo2Btn = document.querySelector('#go-to-2-slide');
goTo2Btn.addEventListener('click', () => {
const carousel = new HSCarousel(document.querySelector('#carouselTarget'));
carousel.goTo(1);
});
Go to certain slide by button click example (mixed method).
const carousel = HSCarousel.getInstance('#carouselTarget');
const goTo2Btn = document.querySelector('#go-to-2-slide');
goTo2Btn.addEventListener('click', () => {
carousel.goTo(1);
});