third party plugins
Tailwind CSS Drag & Drop
Implement drag-and-drop, reorderable lists for modern browsers and touch devices using Sortable.js.
Below are the comprehensively outlined steps you can follow to seamlessly integrate SortableJs with FlyonUI.
- 1Installation
Install
Install Sortable.js.
using npm:npm install sortablejs
- 2Include SortableJs JavaScript
Include the following JS files in your page:
<body> <script src="../path/to/vendor/sortablejs/Sortable.min.js"></script> </body>
- 3Initialize SortableJs
Add the SortableJS initialization JavaScript code below the SortableJS JS library inclusion. Set up the Sortable instance to target elements using their
id
attribute.const example1 = document.querySelector('#example') Sortable.create(example1, { animation: 150, });
Here’s how a basic drag-and-drop list appears with the simplest markup.
- Weekly Insights
- Resource Center
- Team Collaboration
- Product Updates
- Community Forum
<ul id="list-example" class="border-base-content/25 divide-base-content/25 flex flex-col divide-y rounded-md border *:cursor-move *:p-3 first:*:rounded-t-md last:*:rounded-b-md" >
<li class="flex items-center gap-3">
<span class="icon-[tabler--bell] size-4 shrink-0"></span>
Weekly Insights
<span class="icon-[tabler--grip-vertical] text-base-content ms-auto size-4 shrink-0"></span>
</li>
<li class="flex items-center gap-3">
<span class="icon-[tabler--cloud-download] size-4 shrink-0"></span>
Resource Center
<span class="icon-[tabler--grip-vertical] text-base-content ms-auto size-4 shrink-0"></span>
</li>
<li class="flex items-center gap-3">
<span class="icon-[tabler--users] size-4 shrink-0"></span>
Team Collaboration
<span class="icon-[tabler--grip-vertical] text-base-content ms-auto size-4 shrink-0"></span>
</li>
<li class="flex items-center gap-3">
<span class="icon-[tabler--bell] size-4 shrink-0"></span>
Product Updates
<span class="icon-[tabler--grip-vertical] text-base-content ms-auto size-4 shrink-0"></span>
</li>
<li class="flex items-center gap-3">
<span class="icon-[tabler--users] size-4 shrink-0"></span>
Community Forum
<span class="icon-[tabler--grip-vertical] text-base-content ms-auto size-4 shrink-0"></span>
</li>
</ul>
<script>
window.addEventListener('load', () => {
;(function () {
// Basic example
const listExample = document.querySelector('#list-example')
if (listExample) {
Sortable.create(listExample, {
animation: 150,
dragClass: '!border-0'
})
}
})()
})
</script>
These stats represent a real-time overview of key business metrics, including orders, revenue, invoices, and shipments
<div id="stat-example" class="grid grid-cols-2 gap-6 *:cursor-move">
<div class="stats max-sm:w-full">
<div class="stat">
<div class="avatar placeholder">
<div class="bg-success/20 text-success size-10 rounded-full">
<span class="icon-[tabler--package] size-6"></span>
</div>
</div>
<div class="stat-value mb-1">Order</div>
<div class="stat-title">7,500 of 10,000 orders</div>
<div class="progress bg-success/10 h-2" role="progressbar" aria-label="Order Progressbar" aria-valuenow="75" aria-valuemin="0" aria-valuemax="100" >
<div class="progress-bar progress-success w-3/4"></div>
</div>
</div>
</div>
<div class="stats max-sm:w-full">
<div class="stat">
<div class="avatar placeholder">
<div class="bg-warning/20 text-warning size-10 rounded-full">
<span class="icon-[tabler--cash] size-6"></span>
</div>
</div>
<div class="stat-value mb-1">Revenue</div>
<div class="stat-title">$45,000 of $100,000</div>
<div class="progress bg-warning/10 h-2" role="progressbar" aria-label="Revenue Progressbar" aria-valuenow="45" aria-valuemin="0" aria-valuemax="100" >
<div class="progress-bar progress-warning w-2/5"></div>
</div>
</div>
</div>
<div class="stats max-sm:w-full">
<div class="stat">
<div class="avatar placeholder">
<div class="bg-error/20 text-error size-10 rounded-full">
<span class="icon-[tabler--credit-card] size-6"></span>
</div>
</div>
<div class="stat-value mb-1">Invoice</div>
<div class="stat-title">$18,200 of $25,000</div>
<div class="progress bg-error/10 h-2" role="progressbar" aria-label="Invoice Progressbar" aria-valuenow="73" aria-valuemin="0" aria-valuemax="100" >
<div class="progress-bar progress-error w-9/12"></div>
</div>
</div>
</div>
<!-- Shipment Stats -->
<div class="stats">
<div class="stat">
<div class="avatar placeholder">
<div class="bg-info/20 text-info size-10 rounded-full">
<span class="icon-[tabler--truck] size-6"></span>
</div>
</div>
<div class="stat-value mb-1">Shipments</div>
<div class="stat-title">10,450 of 12,000 shipments</div>
<div class="progress bg-info/10 h-2" role="progressbar" aria-label="Invoice Progressbar" aria-valuenow="73" aria-valuemin="0" aria-valuemax="100" >
<div class="progress-bar progress-info w-11/12"></div>
</div>
</div>
</div>
</div>
<script>
window.addEventListener('load', () => {
;(function () {
// Stat example
const statExample = document.querySelector('#stat-example')
if (statExample) {
Sortable.create(statExample, {
animation: 150
})
}
})()
})
</script>
This showcases a list of avatars with a drag-and-drop interaction, perfect for user selection or grouping.
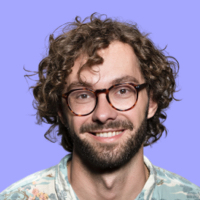
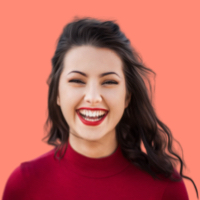
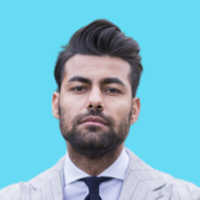
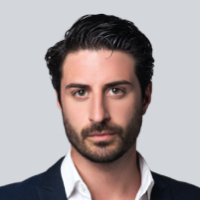
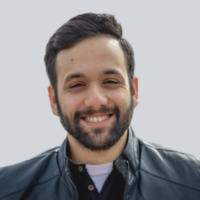
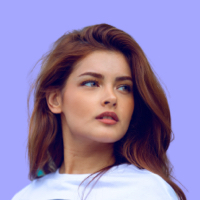
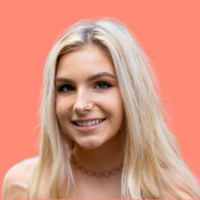
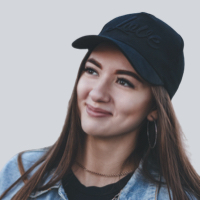
<div class="flew-wrap flex items-center gap-3 *:cursor-move" id="image-example">
<div class="avatar">
<div class="size-10 rounded-full">
<img src="https://cdn.flyonui.com/fy-assets/avatar/avatar-1.png" alt="avatar" />
</div>
</div>
<div class="avatar">
<div class="size-10 rounded-full">
<img src="https://cdn.flyonui.com/fy-assets/avatar/avatar-2.png" alt="avatar" />
</div>
</div>
<div class="avatar">
<div class="size-10 rounded-full">
<img src="https://cdn.flyonui.com/fy-assets/avatar/avatar-3.png" alt="avatar" />
</div>
</div>
<div class="avatar">
<div class="size-10 rounded-full">
<img src="https://cdn.flyonui.com/fy-assets/avatar/avatar-5.png" alt="avatar" />
</div>
</div>
<div class="avatar">
<div class="size-10 rounded-full">
<img src="https://cdn.flyonui.com/fy-assets/avatar/avatar-7.png" alt="avatar" />
</div>
</div>
<div class="avatar">
<div class="size-10 rounded-full">
<img src="https://cdn.flyonui.com/fy-assets/avatar/avatar-10.png" alt="avatar" />
</div>
</div>
<div class="avatar">
<div class="size-10 rounded-full">
<img src="https://cdn.flyonui.com/fy-assets/avatar/avatar-12.png" alt="avatar" />
</div>
</div>
<div class="avatar">
<div class="size-10 rounded-full">
<img src="https://cdn.flyonui.com/fy-assets/avatar/avatar-15.png" alt="avatar" />
</div>
</div>
</div>
<script>
window.addEventListener('load', () => {
;(function () {
// Image example
const imageExample = document.querySelector('#image-example')
if (imageExample) {
Sortable.create(imageExample, {
animation: 150
})
}
})()
})
</script>
Example of a shared task list with ‘Pending’ and ‘Completed’ tasks, each assigned to a user with an avatar.
Pending Tasks
- Design new company logo.
- Prepare quarterly report.
- Schedule team meeting.
- Update client database.
- Plan marketing campaign.
Completed Tasks
- Launch new website.
- Finalize budget proposal.
- Conduct employee training.
- Organize office relocation.
- Attend industry conference.
<div class="grid grid-cols-1 gap-5 sm:grid-cols-2 p-4 bg-base-100 shadow rounded-box w-full">
<div>
<p class="text-base-content text-base font-semibold">Pending Tasks</p>
<ul class="divide-base-content/25 divide-y *:cursor-move *:p-3 *:flex *:items-center" id="pending-tasks">
<li>
<span>Design new company logo.</span>
<div class="avatar ms-auto">
<div class="size-6 rounded-full">
<img src="https://cdn.flyonui.com/fy-assets/avatar/avatar-11.png" alt="avatar" />
</div>
</div>
</li>
<li>
<span>Prepare quarterly report.</span>
<div class="avatar ms-auto">
<div class="size-6 rounded-full">
<img src="https://cdn.flyonui.com/fy-assets/avatar/avatar-12.png" alt="avatar" />
</div>
</div>
</li>
<li>
<span>Schedule team meeting.</span>
<div class="avatar ms-auto">
<div class="size-6 rounded-full">
<img src="https://cdn.flyonui.com/fy-assets/avatar/avatar-13.png" alt="avatar" />
</div>
</div>
</li>
<li>
<span>Update client database.</span>
<div class="avatar ms-auto">
<div class="size-6 rounded-full">
<img src="https://cdn.flyonui.com/fy-assets/avatar/avatar-14.png" alt="avatar" />
</div>
</div>
</li>
<li>
<span>Plan marketing campaign.</span>
<div class="avatar ms-auto">
<div class="size-6 rounded-full">
<img src="https://cdn.flyonui.com/fy-assets/avatar/avatar-15.png" alt="avatar" />
</div>
</div>
</li>
</ul>
</div>
<div>
<p class="text-base-content text-base font-semibold">Completed Tasks</p>
<ul class="divide-base-content/25 divide-y *:cursor-move *:p-3 *:flex *:items-center" id="completed-tasks">
<li>
<span>Launch new website.</span>
<div class="avatar ms-auto">
<div class="size-6 rounded-full">
<img src="https://cdn.flyonui.com/fy-assets/avatar/avatar-16.png" alt="avatar" />
</div>
</div>
</li>
<li>
<span>Finalize budget proposal.</span>
<div class="avatar ms-auto">
<div class="size-6 rounded-full">
<img src="https://cdn.flyonui.com/fy-assets/avatar/avatar-17.png" alt="avatar" />
</div>
</div>
</li>
<li>
<span>Conduct employee training.</span>
<div class="avatar ms-auto">
<div class="size-6 rounded-full">
<img src="https://cdn.flyonui.com/fy-assets/avatar/avatar-18.png" alt="avatar" />
</div>
</div>
</li>
<li>
<span>Organize office relocation.</span>
<div class="avatar ms-auto">
<div class="size-6 rounded-full">
<img src="https://cdn.flyonui.com/fy-assets/avatar/avatar-19.png" alt="avatar" />
</div>
</div>
</li>
<li>
<span>Attend industry conference.</span>
<div class="avatar ms-auto">
<div class="size-6 rounded-full">
<img src="https://cdn.flyonui.com/fy-assets/avatar/avatar-20.png" alt="avatar" />
</div>
</div>
</li>
</ul>
</div>
</div>
<script>
window.addEventListener('load', () => {
;(function () {
// shared example
const pendingTasks = document.querySelector('#pending-tasks')
const completedTasks = document.querySelector('#completed-tasks')
if (pendingTasks) {
Sortable.create(pendingTasks, {
animation: 150,
group: 'taskList',
dragClass: '!border-0'
})
}
if (completedTasks) {
Sortable.create(completedTasks, {
animation: 150,
group: 'taskList',
dragClass: '!border-0'
})
}
})()
})
</script>
Example of a cloned task list with ‘Pending Tasks’ and ‘Completed Tasks’, each task assigned to a user with an avatar."
Pending Tasks
- Develop mobile app prototype.
- Research market trends.
- Organize product launch event.
- Update company policy documents.
- Design promotional materials.
- Prepare investor pitch deck.
Completed Tasks
- Complete quarterly audit.
- Launch social media campaign.
- Train new staff members.
- Upgrade office equipment.
- Submit project deliverables.
- Host client appreciation dinner.
<div class="bg-base-100 rounded-box grid w-full grid-cols-1 gap-5 p-4 shadow sm:grid-cols-2">
<div>
<p class="text-base-content text-base font-semibold">Pending Tasks</p>
<ul class="divide-base-content/25 divide-y *:flex *:cursor-move *:items-center *:p-3" id="clone-source-1">
<li>
<span>Develop mobile app prototype.</span>
<div class="avatar ms-auto">
<div class="size-6 rounded-full">
<img src="https://cdn.flyonui.com/fy-assets/avatar/avatar-21.png" alt="avatar" />
</div>
</div>
</li>
<li>
<span>Research market trends.</span>
<div class="avatar ms-auto">
<div class="size-6 rounded-full">
<img src="https://cdn.flyonui.com/fy-assets/avatar/avatar-22.png" alt="avatar" />
</div>
</div>
</li>
<li>
<span>Organize product launch event.</span>
<div class="avatar ms-auto">
<div class="size-6 rounded-full">
<img src="https://cdn.flyonui.com/fy-assets/avatar/avatar-23.png" alt="avatar" />
</div>
</div>
</li>
<li>
<span>Update company policy documents.</span>
<div class="avatar ms-auto">
<div class="size-6 rounded-full">
<img src="https://cdn.flyonui.com/fy-assets/avatar/avatar-24.png" alt="avatar" />
</div>
</div>
</li>
<li>
<span>Design promotional materials.</span>
<div class="avatar ms-auto">
<div class="size-6 rounded-full">
<img src="https://cdn.flyonui.com/fy-assets/avatar/avatar-25.png" alt="avatar" />
</div>
</div>
</li>
<li>
<span>Prepare investor pitch deck.</span>
<div class="avatar ms-auto">
<div class="size-6 rounded-full">
<img src="https://cdn.flyonui.com/fy-assets/avatar/avatar-26.png" alt="avatar" />
</div>
</div>
</li>
</ul>
</div>
<div>
<p class="text-base-content text-base font-semibold">Completed Tasks</p>
<ul class="divide-base-content/25 divide-y *:flex *:cursor-move *:items-center *:p-3" id="clone-source-2">
<li>
<span>Complete quarterly audit.</span>
<div class="avatar ms-auto">
<div class="size-6 rounded-full">
<img src="https://cdn.flyonui.com/fy-assets/avatar/avatar-29.png" alt="avatar" />
</div>
</div>
</li>
<li>
<span>Launch social media campaign.</span>
<div class="avatar ms-auto">
<div class="size-6 rounded-full">
<img src="https://cdn.flyonui.com/fy-assets/avatar/avatar-8.png" alt="avatar" />
</div>
</div>
</li>
<li>
<span>Train new staff members.</span>
<div class="avatar ms-auto">
<div class="size-6 rounded-full">
<img src="https://cdn.flyonui.com/fy-assets/avatar/avatar-6.png" alt="avatar" />
</div>
</div>
</li>
<li>
<span>Upgrade office equipment.</span>
<div class="avatar ms-auto">
<div class="size-6 rounded-full">
<img src="https://cdn.flyonui.com/fy-assets/avatar/avatar-14.png" alt="avatar" />
</div>
</div>
</li>
<li>
<span>Submit project deliverables.</span>
<div class="avatar ms-auto">
<div class="size-6 rounded-full">
<img src="https://cdn.flyonui.com/fy-assets/avatar/avatar-18.png" alt="avatar" />
</div>
</div>
</li>
<li>
<span>Host client appreciation dinner.</span>
<div class="avatar ms-auto">
<div class="size-6 rounded-full">
<img src="https://cdn.flyonui.com/fy-assets/avatar/avatar-7.png" alt="avatar" />
</div>
</div>
</li>
</ul>
</div>
</div>
<script>
window.addEventListener('load', () => {
;(function () {
// Clone example
(cloneSource1 = document.getElementById('clone-source-1')),
(cloneSource2 = document.getElementById('clone-source-2'))
if (cloneSource1) {
Sortable.create(cloneSource1, {
animation: 150,
group: {
name: 'cloneList',
pull: 'clone',
revertClone: true
},
dragClass: '!border-0'
})
}
if (cloneSource2) {
Sortable.create(cloneSource2, {
animation: 150,
group: {
name: 'cloneList',
pull: 'clone',
revertClone: true
},
dragClass: '!border-0'
})
}
})()
})
</script>
Try sorting the pending tasks. It is not possible because it has it’s sort option set to false. However, you can still drag from the list on the pending tast to the list on the completed task.
Pending Tasks
- Compile Q4 financial statements.
- Review vendor contracts.
- Test new software deployment.
- Create new onboarding materials.
- Analyze customer satisfaction survey.
Completed Tasks
- Launch internal newsletter.
- Finalize project timeline for new product.
- Conduct annual security audit.
- Hold Q3 review meeting with stakeholders.
- Complete brand style guide.
- Update remote work policy.
<div class="bg-base-100 rounded-box grid w-full grid-cols-1 gap-5 p-4 shadow sm:grid-cols-2">
<div>
<p class="text-base-content text-base font-semibold">Pending Tasks</p>
<ul class="divide-base-content/25 divide-y *:flex *:cursor-move *:items-center *:p-3" id="disabled-source-1">
<li>
<span>Compile Q4 financial statements.</span>
<span class="icon-[tabler--grip-vertical] text-base-content ms-auto size-4 shrink-0"></span>
</li>
<li>
<span>Review vendor contracts.</span>
<span class="icon-[tabler--grip-vertical] text-base-content ms-auto size-4 shrink-0"></span>
</li>
<li>
<span>Test new software deployment.</span>
<span class="icon-[tabler--grip-vertical] text-base-content ms-auto size-4 shrink-0"></span>
</li>
<li>
<span>Create new onboarding materials.</span>
<span class="icon-[tabler--grip-vertical] text-base-content ms-auto size-4 shrink-0"></span>
</li>
<li>
<span>Analyze customer satisfaction survey.</span>
<span class="icon-[tabler--grip-vertical] text-base-content ms-auto size-4 shrink-0"></span>
</li>
</ul>
</div>
<div>
<p class="text-base-content text-base font-semibold">Completed Tasks</p>
<ul class="divide-base-content/25 divide-y *:flex *:cursor-move *:items-center *:p-3" id="disabled-source-2">
<li>
<span>Launch internal newsletter.</span>
<span class="icon-[tabler--grip-vertical] text-base-content ms-auto size-4 shrink-0"></span>
</li>
<li>
<span>Finalize project timeline for new product.</span>
<span class="icon-[tabler--grip-vertical] text-base-content ms-auto size-4 shrink-0"></span>
</li>
<li>
<span>Conduct annual security audit.</span>
<span class="icon-[tabler--grip-vertical] text-base-content ms-auto size-4 shrink-0"></span>
</li>
<li>
<span>Hold Q3 review meeting with stakeholders.</span>
<span class="icon-[tabler--grip-vertical] text-base-content ms-auto size-4 shrink-0"></span>
</li>
<li>
<span>Complete brand style guide.</span>
<span class="icon-[tabler--grip-vertical] text-base-content ms-auto size-4 shrink-0"></span>
</li>
<li>
<span>Update remote work policy.</span>
<span class="icon-[tabler--grip-vertical] text-base-content ms-auto size-4 shrink-0"></span>
</li>
</ul>
</div>
</div>
<script>
window.addEventListener('load', () => {
;(function () {
// disabled example
;(disabledSource1 = document.getElementById('disabled-source-1')),
(disabledSource2 = document.getElementById('disabled-source-2'))
if (disabledSource1) {
Sortable.create(disabledSource1, {
animation: 150,
group: {
name: 'disabledList',
pull: 'clone',
put: false // Do not allow items to be put into this list
},
sort: false, // To disable sorting: set sort to false
dragClass: '!border-0'
})
}
if (disabledSource2) {
Sortable.create(disabledSource2, {
animation: 150,
group: 'disabledList',
dragClass: '!border-0'
})
}
})()
})
</script>
Example of a list where items can be rearranged using a draggable handle, allowing only the handle to be used for drag-and-drop functionality.
- Weekly Insights
- Resource Center
- Team Collaboration
- Product Updates
- Community Forum
<ul id="handle-example" class="border-base-content/25 divide-base-content/25 flex flex-col divide-y rounded-md border *:p-3 first:*:rounded-t-md last:*:rounded-b-md" >
<li class="flex items-center gap-3">
<span class="icon-[tabler--arrows-move] text-base-content handle me-1.5 size-4 shrink-0 cursor-move"></span>
<span class="icon-[tabler--bell] size-4 shrink-0"></span>
Weekly Insights
</li>
<li class="flex items-center gap-3">
<span class="icon-[tabler--arrows-move] text-base-content handle me-1.5 size-4 shrink-0 cursor-move"></span>
<span class="icon-[tabler--cloud-download] size-4 shrink-0"></span>
Resource Center
</li>
<li class="flex items-center gap-3">
<span class="icon-[tabler--arrows-move] text-base-content handle me-1.5 size-4 shrink-0 cursor-move"></span>
<span class="icon-[tabler--users] size-4 shrink-0"></span>
Team Collaboration
</li>
<li class="flex items-center gap-3">
<span class="icon-[tabler--arrows-move] text-base-content handle me-1.5 size-4 shrink-0 cursor-move"></span>
<span class="icon-[tabler--bell] size-4 shrink-0"></span>
Product Updates
</li>
<li class="flex items-center gap-3">
<span class="icon-[tabler--arrows-move] text-base-content handle me-1.5 size-4 shrink-0 cursor-move"></span>
<span class="icon-[tabler--users] size-4 shrink-0"></span>
Community Forum
</li>
</ul>
<script>
window.addEventListener('load', () => {
;(function () {
// Handle example
const handleExample = document.querySelector('#handle-example')
if (handleExample) {
Sortable.create(handleExample, {
animation: 150,
dragClass: '!border-0',
handle: '.handle' // handle's class
})
}
})()
})
</script>
Example of a nested list where you can drag and drop items to reorder them, even within different levels. Each item has a handle for easy dragging and rearranging.
fallbackOnBody
option to true
. Additionally, it's recommended to either set the invertSwap
option to true
or reduce the swapThreshold
to a value lower than the default (e.g., 0.65).<div id="nested-sortable" class="w-full">
<div class="nested-sortable-item space-y-1">
<div class="nested-1 space-y-1">
<div class="p-3 flex items-center gap-x-3 cursor-move bg-base-100 border border-base-content/25 rounded-lg font-medium text-sm text-base-content/80">
Item 1.1
<span class="icon-[tabler--grip-vertical] text-base-content ms-auto size-4 shrink-0"></span>
</div>
<div class="ps-5 space-y-1 nested-sortable-item">
<div class="nested-2">
<div class="p-3 flex items-center gap-x-3 cursor-move bg-base-200/60 border border-base-content/25 rounded-lg font-medium text-sm text-base-content/80">
Item 2.1
<span class="icon-[tabler--grip-vertical] text-base-content ms-auto size-4 shrink-0"></span>
</div>
</div>
<div class="nested-2 space-y-1">
<div class="p-3 flex items-center gap-x-3 cursor-move bg-base-200/60 border border-base-content/25 rounded-lg font-medium text-sm text-base-content/80">
Item 2.2
<span class="icon-[tabler--grip-vertical] text-base-content ms-auto size-4 shrink-0"></span>
</div>
<div class="ps-5 space-y-1 nested-sortable-item">
<div class="nested-3">
<div class="p-3 flex items-center gap-x-3 cursor-move bg-base-200 border border-base-content/25 rounded-lg font-medium text-sm text-base-content/80">
Item 3.1
<span class="icon-[tabler--grip-vertical] text-base-content ms-auto size-4 shrink-0"></span>
</div>
</div>
<div class="nested-3">
<div class="p-3 flex items-center gap-x-3 cursor-move bg-base-200 border border-base-content/25 rounded-lg font-medium text-sm text-base-content/80">
Item 3.2
<span class="icon-[tabler--grip-vertical] text-base-content ms-auto size-4 shrink-0"></span>
</div>
</div>
<div class="nested-3">
<div class="p-3 flex items-center gap-x-3 cursor-move bg-base-200 border border-base-content/25 rounded-lg font-medium text-sm text-base-content/80">
Item 3.3
<span class="icon-[tabler--grip-vertical] text-base-content ms-auto size-4 shrink-0"></span>
</div>
</div>
<div class="nested-3">
<div class="p-3 flex items-center gap-x-3 cursor-move bg-base-200 border border-base-content/25 rounded-lg font-medium text-sm text-base-content/80">
Item 3.4
<span class="icon-[tabler--grip-vertical] text-base-content ms-auto size-4 shrink-0"></span>
</div>
</div>
</div>
</div>
<div class="nested-2">
<div class="p-3 flex items-center gap-x-3 cursor-move bg-base-200/60 border border-base-content/25 rounded-lg font-medium text-sm text-base-content/80">
Item 2.3
<span class="icon-[tabler--grip-vertical] text-base-content ms-auto size-4 shrink-0"></span>
</div>
</div>
<div class="nested-2">
<div class="p-3 flex items-center gap-x-3 cursor-move bg-base-200/60 border border-base-content/25 rounded-lg font-medium text-sm text-base-content/80">
Item 2.4
<span class="icon-[tabler--grip-vertical] text-base-content ms-auto size-4 shrink-0"></span>
</div>
</div>
</div>
</div>
<div class="nested-1">
<div class="p-3 flex items-center gap-x-3 cursor-move bg-base-100 border border-base-content/25 rounded-lg font-medium text-sm text-base-content/80">
Item 1.2
<span class="icon-[tabler--grip-vertical] text-base-content ms-auto size-4 shrink-0"></span>
</div>
</div>
<div class="nested-1">
<div class="p-3 flex items-center gap-x-3 cursor-move bg-base-100 border border-base-content/25 rounded-lg font-medium text-sm text-base-content/80">
Item 1.3
<span class="icon-[tabler--grip-vertical] text-base-content ms-auto size-4 shrink-0"></span>
</div>
</div>
<div class="nested-1 space-y-1">
<div class="p-3 flex items-center gap-x-3 cursor-move bg-base-100 border border-base-content/25 rounded-lg font-medium text-sm text-base-content/80">
Item 1.4
<span class="icon-[tabler--grip-vertical] text-base-content ms-auto size-4 shrink-0"></span>
</div>
<div class="ps-5 space-y-1 nested-sortable-item">
<div class="nested-2">
<div class="p-3 flex items-center gap-x-3 cursor-move bg-base-200/60 border border-base-content/25 rounded-lg font-medium text-sm text-base-content/80">
Item 2.1
<span class="icon-[tabler--grip-vertical] text-base-content ms-auto size-4 shrink-0"></span>
</div>
</div>
<div class="nested-2">
<div class="p-3 flex items-center gap-x-3 cursor-move bg-base-200/60 border border-base-content/25 rounded-lg font-medium text-sm text-base-content/80">
Item 2.2
<span class="icon-[tabler--grip-vertical] text-base-content ms-auto size-4 shrink-0"></span>
</div>
</div>
<div class="nested-2">
<div class="p-3 flex items-center gap-x-3 cursor-move bg-base-200/60 border border-base-content/25 rounded-lg font-medium text-sm text-base-content/80">
Item 2.3
<span class="icon-[tabler--grip-vertical] text-base-content ms-auto size-4 shrink-0"></span>
</div>
</div>
<div class="nested-2">
<div class="p-3 flex items-center gap-x-3 cursor-move bg-base-200/60 border border-base-content/25 rounded-lg font-medium text-sm text-base-content/80">
Item 2.4
<span class="icon-[tabler--grip-vertical] text-base-content ms-auto size-4 shrink-0"></span>
</div>
</div>
</div>
</div>
<div class="nested-1">
<div class="p-3 flex items-center gap-x-3 cursor-move bg-base-100 border border-base-content/25 rounded-lg font-medium text-sm text-base-content/80">
Item 1.5
<span class="icon-[tabler--grip-vertical] text-base-content ms-auto size-4 shrink-0"></span>
</div>
</div>
</div>
</div>
<script>
window.addEventListener('load', () => {
;(function () {
// Nested example
const nestedSortables = document.querySelectorAll('#nested-sortable .nested-sortable-item')
for (var i = 0; i < nestedSortables.length; i++) {
Sortable.create(nestedSortables[i], {
group: 'nested',
animation: 150,
fallbackOnBody: true,
swapThreshold: 0.65
})
}
})()
})
</script>
The Swap plugin changes the behaviour of Sortable to allow for items to be swapped with eachother rather than sorted.
- Weekly Insights
- Resource Center
- Team Collaboration
- Product Updates
- Community Forum
<ul id="swap-example" class="border-base-content/25 divide-base-content/25 flex flex-col divide-y rounded-md border *:cursor-move *:p-3 first:*:rounded-t-md last:*:rounded-b-md" >
<li class="flex items-center gap-3">
<span class="icon-[tabler--bell] size-4 shrink-0"></span>
Weekly Insights
<span class="icon-[tabler--grip-vertical] text-base-content ms-auto size-4 shrink-0"></span>
</li>
<li class="flex items-center gap-3">
<span class="icon-[tabler--cloud-download] size-4 shrink-0"></span>
Resource Center
<span class="icon-[tabler--grip-vertical] text-base-content ms-auto size-4 shrink-0"></span>
</li>
<li class="flex items-center gap-3">
<span class="icon-[tabler--users] size-4 shrink-0"></span>
Team Collaboration
<span class="icon-[tabler--grip-vertical] text-base-content ms-auto size-4 shrink-0"></span>
</li>
<li class="flex items-center gap-3">
<span class="icon-[tabler--bell] size-4 shrink-0"></span>
Product Updates
<span class="icon-[tabler--grip-vertical] text-base-content ms-auto size-4 shrink-0"></span>
</li>
<li class="flex items-center gap-3">
<span class="icon-[tabler--users] size-4 shrink-0"></span>
Community Forum
<span class="icon-[tabler--grip-vertical] text-base-content ms-auto size-4 shrink-0"></span>
</li>
</ul>
<script>
window.addEventListener('load', () => {
;(function () {
// Swap example
const swapExample = document.querySelector('#swap-example')
if (swapExample) {
Sortable.create(swapExample, {
animation: 150,
swap: true, // Enable swap plugin
swapClass: '!text-bg-soft-primary', // The class applied to the hovered swap item
dragClass: '!border-0'
})
}
})()
})
</script>