Set up FlyonUI with NextJS using Tailwind CSS
Use FlyonUI with Next.js and Tailwind CSS to design a cutting-edge, responsive interface, optimizing your development experience.
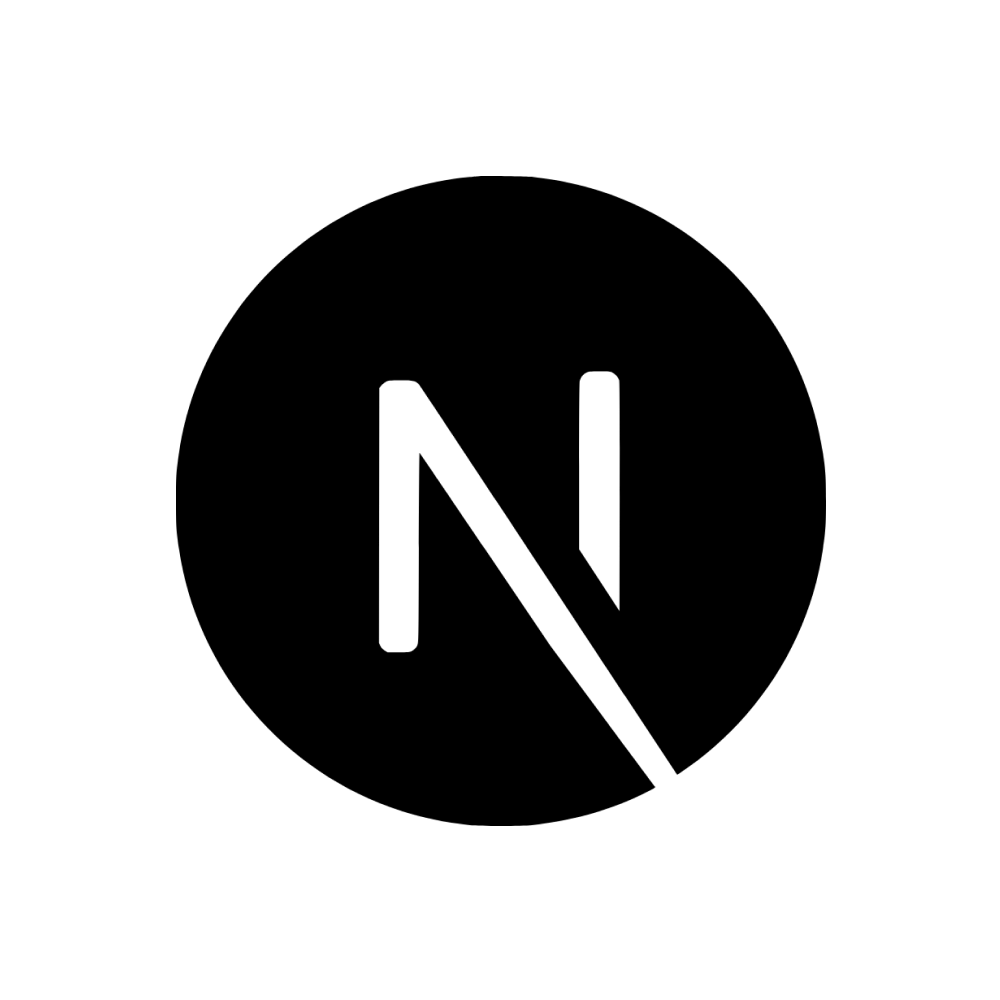
Installation
Please note that the plugin has been tested with the latest version of the framework (v14.2.15). The framework was installed using the standard `npx create-next-app@latest` command. If you are using your own project structure or a different version, pay attention to the file paths and features of your version!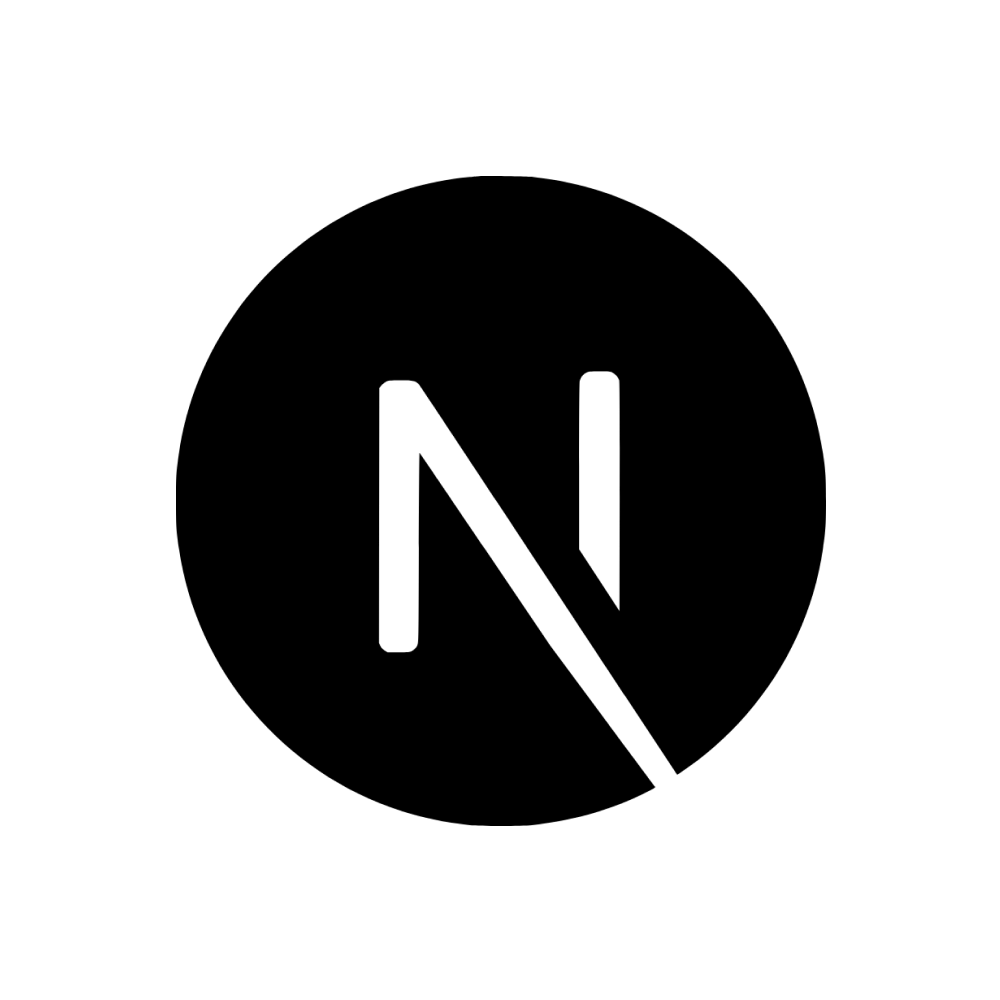
Quick Next.js setup
Next.js is a React framework for server-side rendering and static site generation. If you haven't set up Tailwind CSS yet, Next.js Tailwind CSS check out installation guides.
FlyonUI + Next.js
Explore playground demo on StackBlitz