Set up FlyonUI with Remix using Tailwind CSS
Integrate FlyonUI with Remix and Tailwind CSS to create a modern, responsive UI, streamlining your development process for faster and more efficient results.
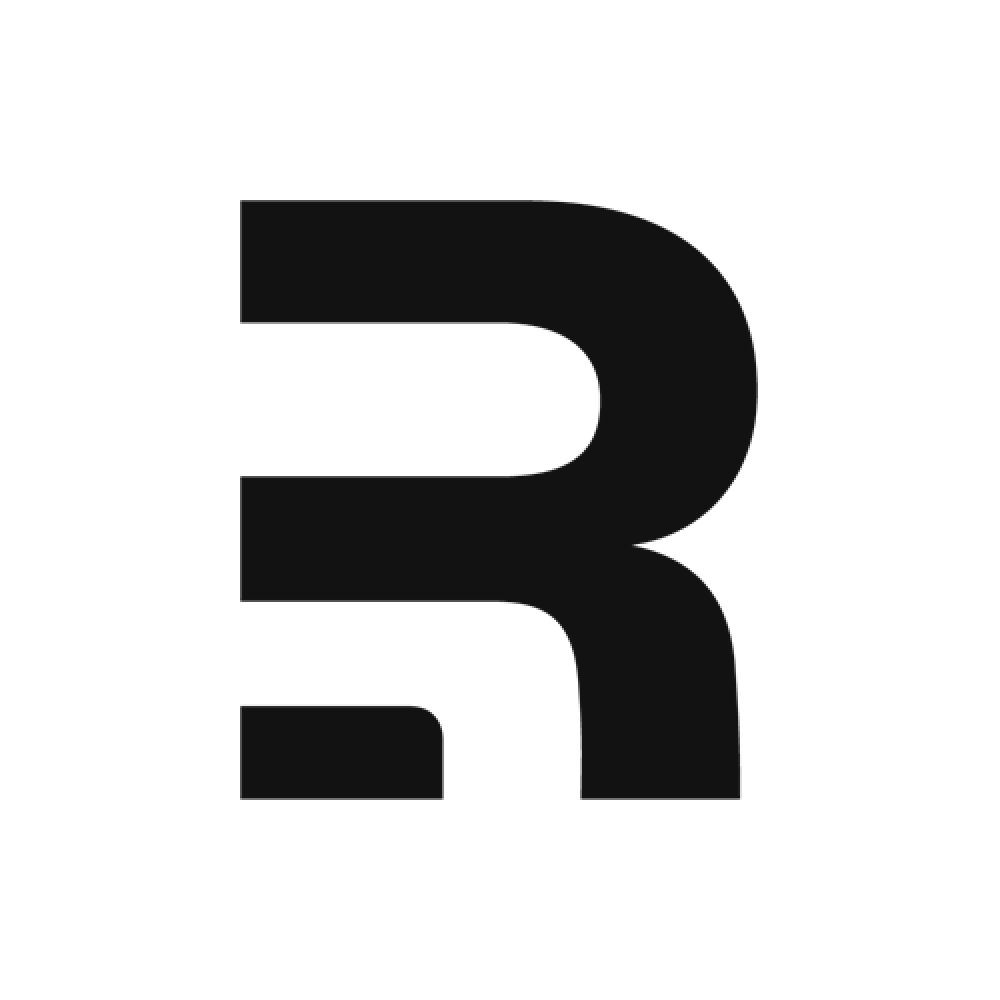
Installation
Please note that the plugin has been tested with the latest version of the framework (v2.13.1). The framework was installed using the standard `npx create-remix@latest project-name` command. If you are using your own project structure or a different version, pay attention to the file paths and features of your version!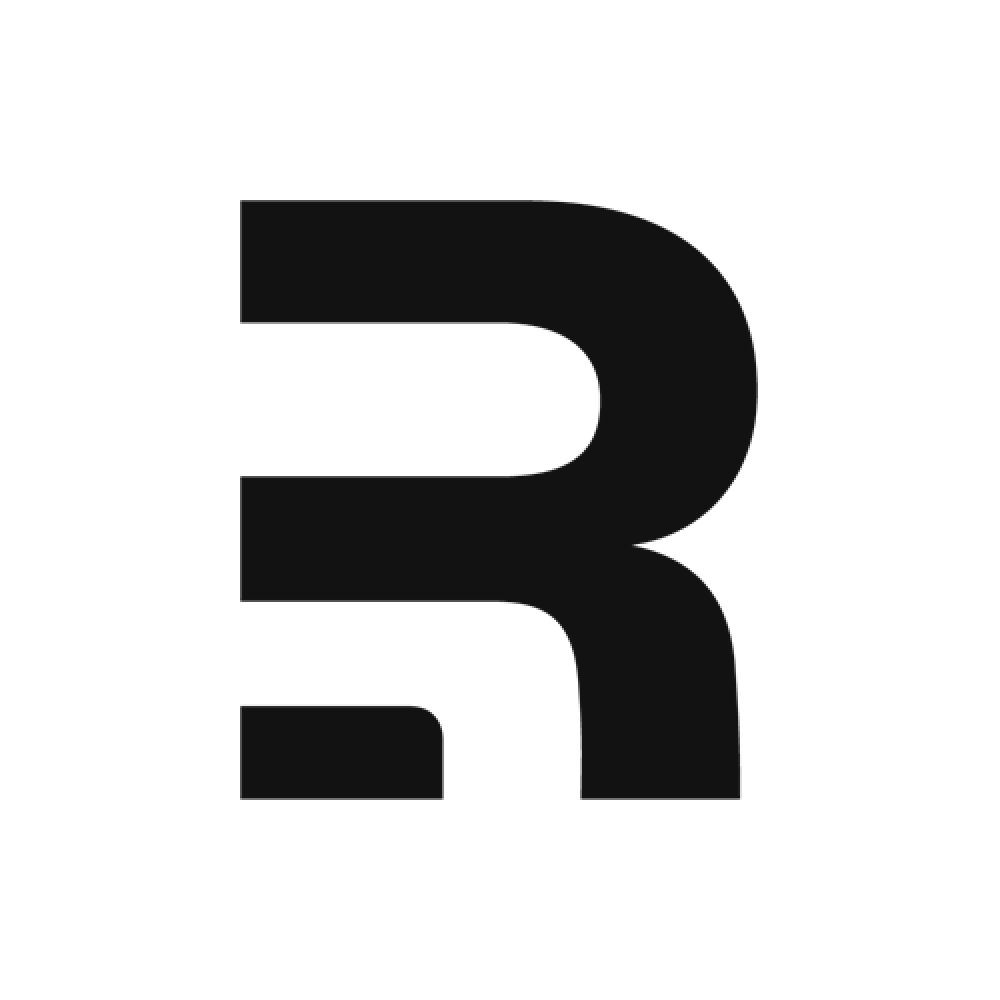
Quick Remix setup
Remix is a full stack React web framework. If you haven't set up Tailwind CSS yet, check out Remix Tailwind CSS installation guides.
FlyonUI + Remix
Explore playground demo on StackBlitz