Set up FlyonUI with SolidJS using Tailwind CSS
Integrate FlyonUI with SolidJS and Tailwind CSS to create a modern, responsive UI, simplifying your development workflow with ease.
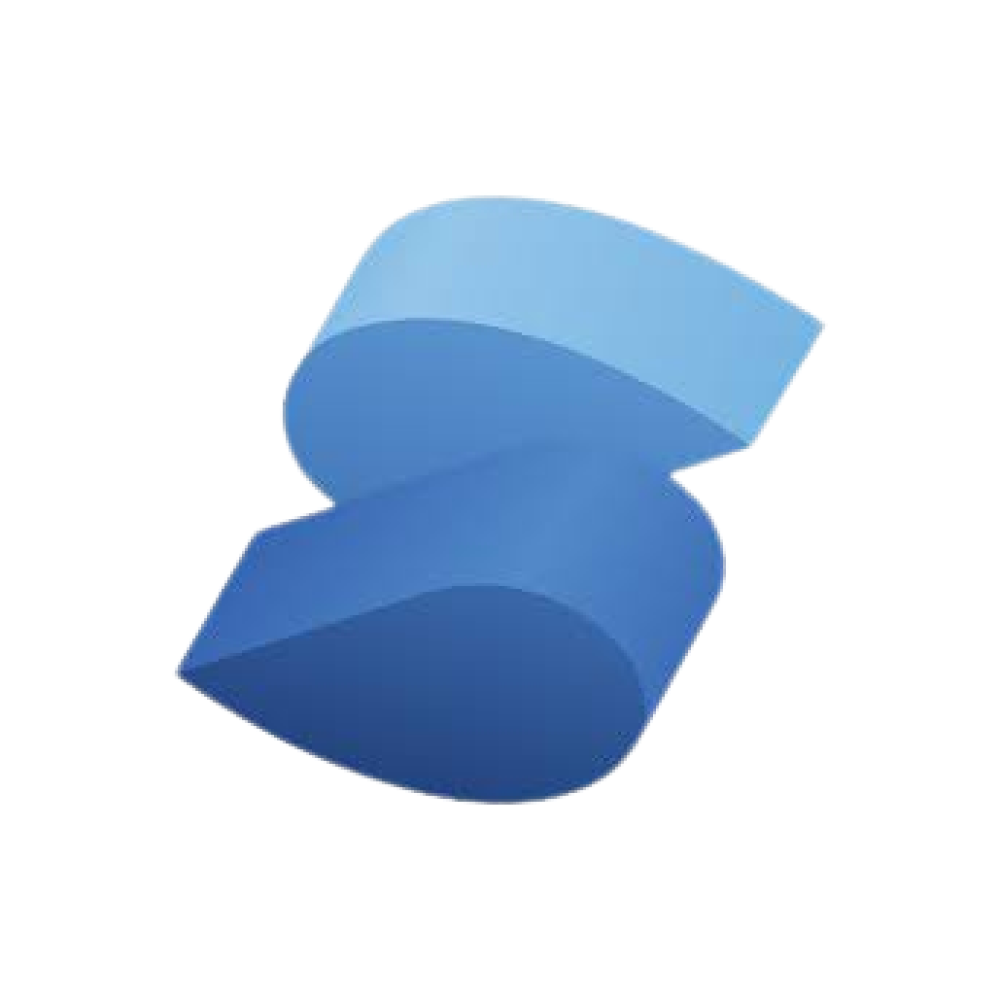
Installation
Please note that the plugin has been tested with the latest version of the framework (v1.9.0). The framework was installed using the standard `npm create vite@latest project-name -- --template solid` command. If you are using your own project structure, be mindful of file paths!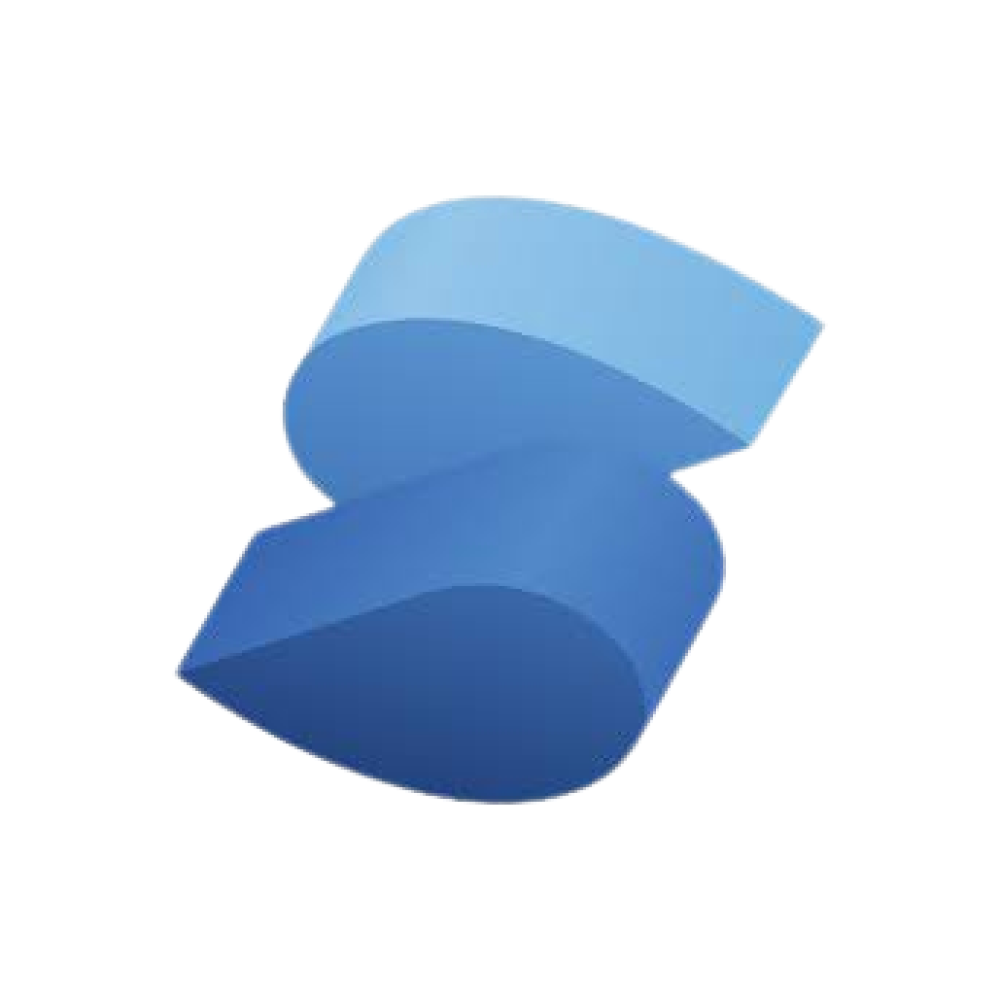
Quick SolidJS setup
A tool for building simple, performant, and reactive user interfaces. If you haven't set up Tailwind CSS yet, check out SolidJS Tailwind CSS installation guides.
FlyonUI + SolidJS
Explore playground demo on StackBlitz